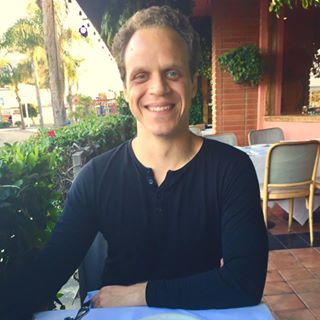
In programming, a constant is a value that will remain immutable. This means that the value stored in it cannot be changed (during the lifecycle of the application). This is different from variables, which are always mutable and whose values can change at any time (hence the name variable).
In Swift, constants are declared using the let keyword, followed by their name. Optionally, one can add the type annotation, containing the type of data the constant represents (an integer, a string, an array, etc.) and then finally the value of that constant. Here is an example:
let backgroundColor = SKColor.black.withAlphaComponent(0.75) // without the type annotation let backgroundColor:SKColor = SKColor.black.withAlphaComponent(0.75) // with type annotation
In iOS, constants are very important for multiple reasons. Primarily, they are used as a safety feature. If one tries to change a constant in code, the XCode compiler will complain instantly, reminding one that one should not try not to change something that one created to remain immutable - a reminder that makes a program much safer against those hard-to-find bugs.
Another reason for using constants is memory. Very often programmers realize in the middle of a project that they are building this one variable every time in several different places, and that variable does not change. Declaring a constant once, and then looking up the reference to that constant, instead of creating a new variable would not only boost memory performance, but also CPU usage, power consumption, etc. Apple recommends these performance tips to better the development process.
Using a struct to declare some constants, and creating objects that take multiple arguments to be initialized can be very efficient, and allow the programmer to create reusable objects. Besides the fact that it is easier to code reusable objects just once, it is also a great way to make an app more efficient.
Consider the code:
struct UIConstants{ // Fonts static let titleFont = UIFont(name: "OCR A", size: 26.0) static let headerFont = UIFont(name: "Cantarell Regular", size: 18.0) static let textFont = UIFont(name: "Inconsolata Regular", size: 14.0) // Colors static let emphasisColor = UIColor.orange static let errorColor = UIColor.red static let defaultTextColor = UIColor.lightGray static let headerTitleColor = UIColor.blue /** Generates and returns the standart header label in a TableView */ static func generateHeaderTitleLabel(text:String) -> UILabel{ // Calculating the Label Size let labelHeight = UIConstants.headerFont!.pointSize + 2.0 let width:CGFloat = 300.0 let headerSize = CGSize(width: width, height: labelHeight) // Preparing the label let label = UILabel(frame: CGRect(origin: CGPoint.zero, size: headerSize)) label.textAlignment = NSTextAlignment.center label.textColor = UIConstants.headerTitleColor label.text = text // Return the label return label } }
Now, when a programmer creates a UITableView, and needs to create titles for each section, the problem can be solved with one line of programming, and the confidence that every other UITableView can use the same solution, which would improve the general consistency of the UI of the app, on top of the performance.
override func tableView(_ tableView: UITableView, viewForHeaderInSection section: Int) -> UIView?{ return UIConstants.generateHeaderTitleLabel(text: "Section Title") }
It is easy to overlook the importance of creating constants, but a well-organized and structured code is often the differencce between a great user experience and buggy apps that hamper users.